Best Way To Create Query String From Javascript Object

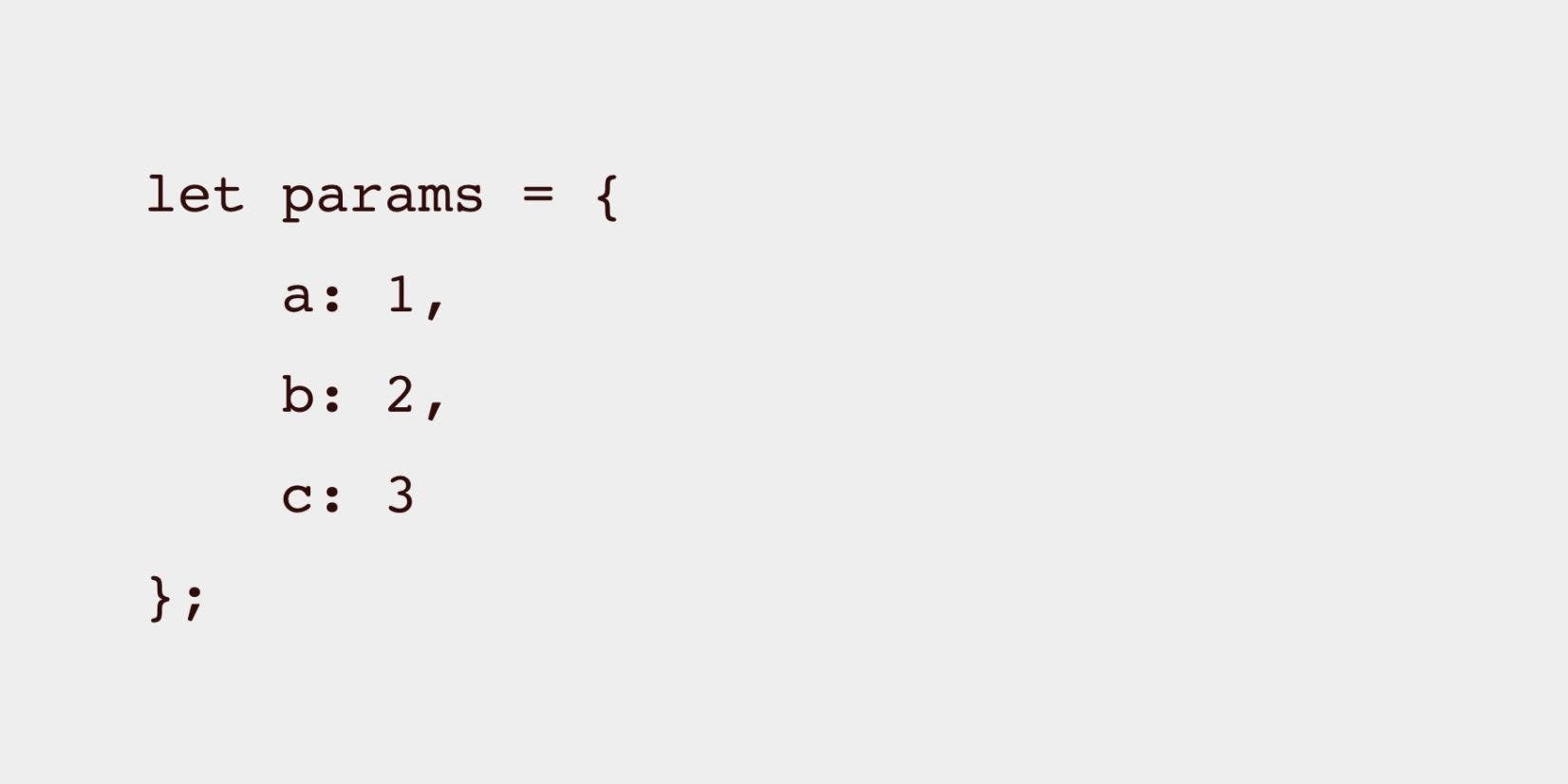

Quite often I find myself building URLs from objects, and if you're developing in JavaScript, you may find this to be a common task as well. Here's what I've found is the best way to create query string from a javascript object.
Basically, we want to be able to take an object like this one:
let params = { a: 1, b: 2, c: 3 };
and convert it into a query string like this:
"a=1&b=2&c=3"
There are a few steps involved in the process, let's review them.
How to create query string from a javascript object:
- Build an Object with known
key: value
pairs for your query string. For values that should have multiple key instances like key[]=1&key[]=2, use an array. - Add any unknown or dynamic values to the Object. Once your initial params object is created you can declare additional key values dynamically or implicitly.
- Map over the Object keys to create an array of encoded strings. The array function
map()
is used onObject.keys()
which creates a new array. UseencodeURIComponent()
function to encode the keys and values correctly. - Join the array of strings to create one query string.
join()
is another array function that we use in tandem withObject.keys()
. - Append the query string and leading question mark to the URL Build your completed URL with encoded URI components.
Whether you know the key/value pairs ahead of time or not , you should use always use encodeURIComponent
to make sure your values translate appropriately and do not break:
// Known parameter values
let params = {
multi: ['one', 'two', '@three'],
utm_source: 'google',
utm_medium: 'cpc',
utm_campaign: 'sale',
code: 'Super Secret $codes'
};
// Example of a value that's dynamic or unknown
params.token = Math.random().toString(36).substring(2, 15) + Math.random().toString(36).substring(2, 15);
// Encode all params with map function on Object keys array,
// assuming value is either string or array
let queryString = Object.keys(params).map((key) => {
if (Array.isArray(params[key])) {
return params[key].map((item, index) => {
return encodeURIComponent(key) + '[' + index + ']=' + encodeURIComponent(item); }).join('&');
} else {
return encodeURIComponent(key) + '=' + encodeURIComponent(params[key])
}
}).join('&');
// Build our url with encoded params string
let url = 'https://example.com/?' + queryString;